4.6. Unit Commitment#
Keywords: semi-continuous variables, cbc usage, gdp, disjunctive programming
4.6.1. Imports#
%matplotlib inline
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
from IPython.display import display, HTML
import shutil
import sys
import os.path
if not shutil.which("pyomo"):
!pip install -q pyomo
assert(shutil.which("pyomo"))
if not (shutil.which("cbc") or os.path.isfile("cbc")):
if "google.colab" in sys.modules:
!apt-get install -y -qq coinor-cbc
else:
try:
!conda install -c conda-forge coincbc
except:
pass
assert(shutil.which("cbc") or os.path.isfile("cbc"))
import pyomo.environ as pyo
import pyomo.gdp as gdp
4.6.2. Problem statement#
A set of \(N\) electrical generating units are available to meet a required demand \(d_t\) for time period \(t \in 1, 2, \ldots, T\). The power generated by unit \(n\) for time period \(t\) is denoted \(x_{n,t}\). Each generating unit is either off, \(x_{n,t} = 0\) or else operating in a range \([p_n^{min}, p_n^{max}]\). The incremental cost of operating the generator during period \(t\) is \(a_n x_{n,t} + b_n\). A binary variable variable \(u_{n,t}\) indicates the operational state of a generating unit.
The unit commitment problem is then
subject to
where we use the short-cut notation \(T = [1, 2, \ldots T]\) and \(N = [1, 2, \ldots, N]\).
This is a minimal model. A realistic model would include additional constraints corresponding to minimum up and down times for generating units, limits on the rate at which power levels can change, maintenance periods, and so forth.
Sun, Xiaoling, Xiaojin Zheng, and Duan Li. “Recent advances in mathematical programming with semi-continuous variables and cardinality constraint.” Journal of the Operations Research Society of China 1, no. 1 (2013): 55-77.
4.6.3. Model#
4.6.3.1. Demand#
# demand
T = 20
T = np.array([t for t in range(0, T)])
d = np.array([100 + 100*np.random.uniform() for t in T])
fig, ax = plt.subplots(1,1)
ax.bar(T+1, d)
ax.set_xlabel('Time Period')
ax.set_title('Demand')
Text(0.5, 1.0, 'Demand')
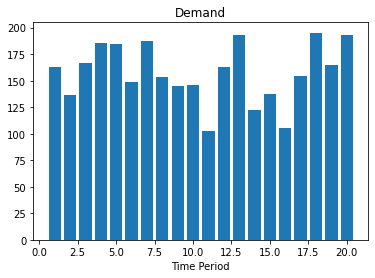
4.6.3.2. Generating Units#
# generating units
N = 5
pmax = 2*max(d)/N
pmin = 0.6*pmax
N = np.array([n for n in range(0, N)])
a = np.array([0.5 + 0.2*np.random.randn() for n in N])
b = np.array([10*np.random.uniform() for n in N])
p = np.linspace(pmin, pmax)
fig, ax = plt.subplots(1,1)
for n in N:
ax.plot(p, a[n]*p + b[n])
ax.set_xlim(0, pmax)
ax.set_ylim(0, max(a*pmax + b))
ax.set_xlabel('Unit Production')
ax.set_ylabel('Unit Operating Cost')
ax.grid()
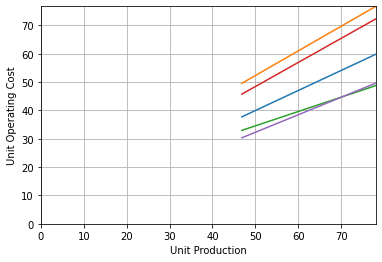
4.6.3.3. Pyomo model 1: Conventional implementation for emi-continuous variables#
def unit_commitment():
m = pyo.ConcreteModel()
m.N = pyo.Set(initialize=N)
m.T = pyo.Set(initialize=T)
m.x = pyo.Var(m.N, m.T, bounds = (0, pmax))
m.u = pyo.Var(m.N, m.T, domain=pyo.Binary)
# objective
m.cost = pyo.Objective(expr = sum(m.x[n,t]*a[n] + m.u[n,t]*b[n] for t in m.T for n in m.N), sense=pyo.minimize)
# demand
m.demand = pyo.Constraint(m.T, rule=lambda m, t: sum(m.x[n,t] for n in N) == d[t])
# semi-continuous
m.lb = pyo.Constraint(m.N, m.T, rule=lambda m, n, t: pmin*m.u[n,t] <= m.x[n,t])
m.ub = pyo.Constraint(m.N, m.T, rule=lambda m, n, t: pmax*m.u[n,t] >= m.x[n,t])
return m
m = unit_commitment()
pyo.SolverFactory('cbc').solve(m).write()
fig, ax = plt.subplots(max(N)+1, 1, figsize=(8, 1.5*max(N)+1))
for n in N:
ax[n].bar(T+1, [m.x[n,t]() for t in T])
ax[n].set_xlim(0, max(T)+2)
ax[n].set_ylim(0, 1.1*pmax)
ax[n].plot(ax[n].get_xlim(), np.array([pmax, pmax]), 'r--')
ax[n].plot(ax[n].get_xlim(), np.array([pmin, pmin]), 'r--')
ax[n].set_title('Unit ' + str(n+1))
fig.tight_layout()
# ==========================================================
# = Solver Results =
# ==========================================================
# ----------------------------------------------------------
# Problem Information
# ----------------------------------------------------------
Problem:
- Name: unknown
Lower bound: 2078.39330587
Upper bound: 2078.39330587
Number of objectives: 1
Number of constraints: 200
Number of variables: 180
Number of binary variables: 100
Number of integer variables: 100
Number of nonzeros: 180
Sense: minimize
# ----------------------------------------------------------
# Solver Information
# ----------------------------------------------------------
Solver:
- Status: ok
User time: -1.0
System time: 0.21
Wallclock time: 0.23
Termination condition: optimal
Termination message: Model was solved to optimality (subject to tolerances), and an optimal solution is available.
Statistics:
Branch and bound:
Number of bounded subproblems: 0
Number of created subproblems: 0
Black box:
Number of iterations: 85
Error rc: 0
Time: 0.2525980472564697
# ----------------------------------------------------------
# Solution Information
# ----------------------------------------------------------
Solution:
- number of solutions: 0
number of solutions displayed: 0
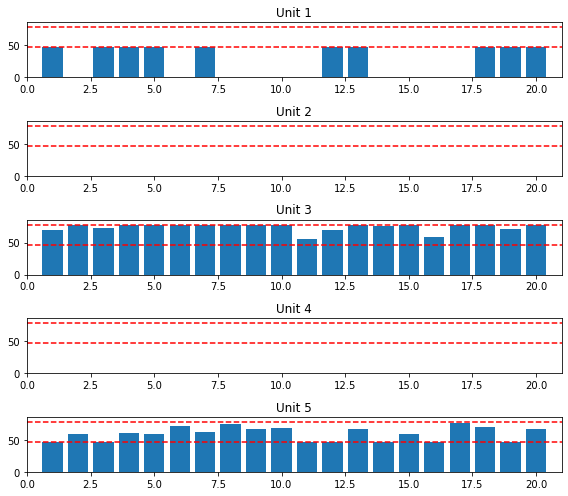
4.6.3.4. Pyomo model 2: GDP implementation#
def unit_commitment_gdp():
m = pyo.ConcreteModel()
m.N = pyo.Set(initialize=N)
m.T = pyo.Set(initialize=T)
m.x = pyo.Var(m.N, m.T, bounds = (0, pmax))
# demand
m.demand = pyo.Constraint(m.T, rule=lambda m, t: sum(m.x[n,t] for n in N) == d[t])
# representing the semicontinous variables as disjuctions
m.sc1 = gdp.Disjunct(m.N, m.T, rule=lambda d, n, t: d.model().x[n,t] == 0)
m.sc2 = gdp.Disjunct(m.N, m.T, rule=lambda d, n, t: d.model().x[n,t] >= pmin)
m.sc = gdp.Disjunction(m.N, m.T, rule=lambda m, n, t: [m.sc1[n,t], m.sc2[n,t]])
# objective. Note use of the disjunct indicator variable
m.cost = pyo.Objective(expr = sum(m.x[n,t]*a[n] + m.sc2[n,t].indicator_var*b[n] for t in m.T for n in m.N), sense=pyo.minimize)
# alternative formulation. But how to access the indicator variable?
#m.semicontinuous = gdp.Disjunction(m.N, m.T, rule=lambda m, n, t: [m.x[n,t]==0, m.x[n,t] >= pmin])
pyo.TransformationFactory('gdp.hull').apply_to(m)
return m
m_gdp = unit_commitment_gdp()
pyo.SolverFactory('cbc').solve(m_gdp).write()
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,0].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,0].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,0].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,0].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,0].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,1].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,1].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,1].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,1].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,1].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,2].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,2].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,2].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,2].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,2].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,3].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,3].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,3].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,3].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,3].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,4].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,4].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,4].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,4].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,4].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,5].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,5].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,5].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,5].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,5].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,6].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,6].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,6].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,6].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,6].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,7].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,7].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,7].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,7].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,7].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,8].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,8].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,8].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,8].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,8].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,9].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,9].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,9].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,9].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,9].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,10].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,10].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,10].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,10].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,10].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,11].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,11].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,11].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,11].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,11].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,12].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,12].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,12].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,12].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,12].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,13].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,13].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,13].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,13].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,13].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,14].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,14].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,14].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,14].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,14].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,15].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,15].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,15].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,15].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,15].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,16].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,16].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,16].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,16].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,16].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,17].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,17].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,17].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,17].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,17].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,18].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,18].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,18].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,18].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,18].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[0,19].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[1,19].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[2,19].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[3,19].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
WARNING: DEPRECATED: Implicit conversion of the Boolean indicator_var
'sc2[4,19].indicator_var' to a binary variable is deprecated and will be
removed. Either express constraints on indicator_var using
LogicalConstraints or work with the associated binary variable from
indicator_var.get_associated_binary() (deprecated in 6.0) (called from /v
ar/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_32621/270333742.p
y:18)
# ==========================================================
# = Solver Results =
# ==========================================================
# ----------------------------------------------------------
# Problem Information
# ----------------------------------------------------------
Problem:
- Name: unknown
Lower bound: 1789.58530805
Upper bound: 1789.58530805
Number of objectives: 1
Number of constraints: 20
Number of variables: 100
Number of binary variables: 200
Number of integer variables: 200
Number of nonzeros: 100
Sense: minimize
# ----------------------------------------------------------
# Solver Information
# ----------------------------------------------------------
Solver:
- Status: ok
User time: -1.0
System time: 0.24
Wallclock time: 0.25
Termination condition: optimal
Termination message: Model was solved to optimality (subject to tolerances), and an optimal solution is available.
Statistics:
Branch and bound:
Number of bounded subproblems: 0
Number of created subproblems: 0
Black box:
Number of iterations: 0
Error rc: 0
Time: 0.28235697746276855
# ----------------------------------------------------------
# Solution Information
# ----------------------------------------------------------
Solution:
- number of solutions: 0
number of solutions displayed: 0
4.6.3.5. There is a problem here!#
Why are the results different? Somehow it appears values of the indicator variables are being ignored.
for n in N:
for t in T:
print("n = {0:2d} t = {1:2d} {2} {3} {4:5.2f}".format(n, t, m_gdp.sc1[n,t].indicator_var(), m_gdp.sc2[n,t].indicator_var(), m.x[n,t]()))
n = 0 t = 0 True False 46.85
n = 0 t = 1 True False 0.00
n = 0 t = 2 True False 46.85
n = 0 t = 3 True False 46.85
n = 0 t = 4 True False 46.85
n = 0 t = 5 True False 0.00
n = 0 t = 6 True False 46.85
n = 0 t = 7 True False 0.00
n = 0 t = 8 True False 0.00
n = 0 t = 9 True False 0.00
n = 0 t = 10 True False 0.00
n = 0 t = 11 True False 46.85
n = 0 t = 12 True False 46.85
n = 0 t = 13 True False 0.00
n = 0 t = 14 True False 0.00
n = 0 t = 15 True False 0.00
n = 0 t = 16 True False 0.00
n = 0 t = 17 True False 46.85
n = 0 t = 18 True False 46.85
n = 0 t = 19 True False 46.85
n = 1 t = 0 True False 0.00
n = 1 t = 1 True False 0.00
n = 1 t = 2 True False 0.00
n = 1 t = 3 True False 0.00
n = 1 t = 4 True False 0.00
n = 1 t = 5 True False 0.00
n = 1 t = 6 True False 0.00
n = 1 t = 7 True False 0.00
n = 1 t = 8 True False 0.00
n = 1 t = 9 True False 0.00
n = 1 t = 10 True False 0.00
n = 1 t = 11 True False 0.00
n = 1 t = 12 True False 0.00
n = 1 t = 13 True False 0.00
n = 1 t = 14 True False 0.00
n = 1 t = 15 True False 0.00
n = 1 t = 16 True False 0.00
n = 1 t = 17 True False 0.00
n = 1 t = 18 True False 0.00
n = 1 t = 19 True False 0.00
n = 2 t = 0 True False 69.26
n = 2 t = 1 True False 78.08
n = 2 t = 2 True False 72.66
n = 2 t = 3 True False 78.08
n = 2 t = 4 True False 78.08
n = 2 t = 5 True False 78.08
n = 2 t = 6 True False 78.08
n = 2 t = 7 True False 78.08
n = 2 t = 8 True False 78.08
n = 2 t = 9 True False 78.08
n = 2 t = 10 True False 56.01
n = 2 t = 11 True False 69.44
n = 2 t = 12 True False 78.08
n = 2 t = 13 True False 75.63
n = 2 t = 14 True False 78.08
n = 2 t = 15 True False 58.14
n = 2 t = 16 True False 78.08
n = 2 t = 17 True False 78.08
n = 2 t = 18 True False 70.69
n = 2 t = 19 True False 78.08
n = 3 t = 0 True False 0.00
n = 3 t = 1 True False 0.00
n = 3 t = 2 True False 0.00
n = 3 t = 3 True False 0.00
n = 3 t = 4 True False 0.00
n = 3 t = 5 True False 0.00
n = 3 t = 6 True False 0.00
n = 3 t = 7 True False 0.00
n = 3 t = 8 True False 0.00
n = 3 t = 9 True False 0.00
n = 3 t = 10 True False 0.00
n = 3 t = 11 True False 0.00
n = 3 t = 12 True False 0.00
n = 3 t = 13 True False 0.00
n = 3 t = 14 True False 0.00
n = 3 t = 15 True False 0.00
n = 3 t = 16 True False 0.00
n = 3 t = 17 True False 0.00
n = 3 t = 18 True False 0.00
n = 3 t = 19 True False 0.00
n = 4 t = 0 True False 46.85
n = 4 t = 1 True False 58.87
n = 4 t = 2 True False 46.85
n = 4 t = 3 True False 60.14
n = 4 t = 4 True False 59.28
n = 4 t = 5 True False 71.02
n = 4 t = 6 True False 62.14
n = 4 t = 7 True False 74.93
n = 4 t = 8 True False 66.69
n = 4 t = 9 True False 67.96
n = 4 t = 10 True False 46.85
n = 4 t = 11 True False 46.85
n = 4 t = 12 True False 67.69
n = 4 t = 13 True False 46.85
n = 4 t = 14 True False 59.46
n = 4 t = 15 True False 46.85
n = 4 t = 16 True False 76.70
n = 4 t = 17 True False 70.27
n = 4 t = 18 True False 46.85
n = 4 t = 19 True False 67.73