Getting Image Data
Contents
Getting Image Data#
The goal of this notebook is to develop a python class for the purpose of counting and labeling flourescent particles captured in images from a prototype for a low-cost medical diagnostic device.
In this notebook we’ll consider traditional image processing techniques .. i.e., those that directly operate on an image to extract scientific information without resort to machine learning techniques. We’ll save that imoportant discussion for another time.
Books and References#
Computer Vision Textbooks
Nixon, Mark, and Alberto Aguado. Feature extraction and image processing for computer vision. Academic press, 2019. link
Szeliski, Richard. Computer vision: algorithms and applications. Springer Science & Business Media, 2010. [Hesburgh Library][Szeliski Web Page and Materials][Preprint of 2nd Edition]
Programming Books
Howse, Joseph, and Joe Minichino. Learning OpenCV 4 Computer Vision with Python 3: Get to grips with tools, techniques, and algorithms for computer vision and machine learning. Packt Publishing Ltd, 2020.
Villán, Alberto Fernández. Mastering OpenCV 4 with Python: A practical guide covering topics from image processing, augmented reality to deep learning with OpenCV 4 and Python 3.7. Packt Publishing Ltd, 2019.
Pajankar, Ashwin. Raspberry Pi Computer Vision Programming: Design and implement computer vision applications with Raspberry Pi, OpenCV, and Python 3. Packt Publishing Ltd, 2020. Amazon
Papers
Coelho, L.P. 2013. Mahotas: Open source software for scriptable computer vision. Journal of Open Research Software 1(1):e3, DOI: http://dx.doi.org/10.5334/jors.ac
Van Noorden, Richard. “Publishers launch joint effort to tackle altered images in research papers.” Nature (2020). https://doi.org/10.1038/d41586-020-01410-9
Blogs and Postings
Digital Camera Sensors for Spectrophotometry
Digital camera sensors commonly employ a color filter array to replicate the
Solli, Martin, et al. “Digital camera characterization for color measurements.” Proceedings of 2005 Beijing International Conference on Imaging: Technology and Applications for the 21st Century. Beijing (China). 2005.
Solli, Martin. Filter characterization in digital cameras. Institutionen för teknik och naturvetenskap, 2004. pdf
Imai, Francisco H., and Roy S. Berns. “Spectral estimation using trichromatic digital cameras.” Proceedings of the International Symposium on Multispectral Imaging and Color Reproduction for Digital Archives. Vol. 42. Chiba University Chiba, Japan, 1999. pdf
Westland, Stephen, Caterina Ripamonti, and Vien Cheung. Computational colour science using MATLAB. John Wiley & Sons, 2012.
Python Packages for Computer Vision#
See https://www.analyticsvidhya.com/blog/2021/04/top-python-libraries-for-image-processing-in-2021/ for a survey of Python libraries for image processing.
Standard Python Libaries
One of the very nice aspects of image processing with Python is the wide adoption of basic NumPy arrays to represent image data. This facilitates the use of methods from multiple packages for a particular project.
NumPy NumPy provides the basic multi-dimensional array data structure used by other image processing libraries.
Matplotlib Matplotlib incudes functions to read image files in several common formats, and display images.
scipy.ndimage SciPy provides a useful collection of image processing algorithms.
scikit-image A library designed to interoperate with NumPy and SciPy.ndimage libraries.
Pure Python Libraries
Pillow A fork of the original Python image library (PIL), Pillow is one of the most important Python libraries for image processing, and the foundation for many other packages. For x86 architectures, Pillow-SIMD is a fork that “follows” Pillow to provide algorithms tuned to the x86 SIMD hardware.
Imageio Imageio is a cross-platform, pure Python library to read and write images, video, volumetric data, and scientific formats. This is a good, lightweight choice if the reading and writing files in multiple formats is the primary purpose.
Full Featured Computer Vision Packages
OpenCV/cv2 OpenCV is a C++ library launched in 1999 at Intel Research to advance real-time computer vision. In 2011 is was taken over by the non-profit foundation OpenCV.org. OpenCV is widely used with mulitple language bindings, full ecosystem of third party documentation, training courses, and books. The Python bindings are accessed by importing the cv2 Python module.
Mahotas A computer vision library written in Python and CPython. While OpenCV is the fastest package, the lack of type checking can result in hard crashes. Mahotas includes type checking with some loss of speed. A paper describing the package is available http://doi.org/10.5334/jors.ac.
SimpleITK The Natonal Library of Medicine Insight Segmentation and Registration Toolkit (ITK). book
Photo Conversion and Management Tools
ImageMagick ImageMagick is a widely-used, full-featured package to create, edit, compose, and convert images. It is available on all major platforms including the Raspberry Pi OS. Wand is a Python binding to the ImageMagick API. See https://www.pythonpool.com/imagemagick-python/ for information using Wand and ImageMagick.
XnView MP A commercial digital asset management tool available on Windows, Mac OS, and Linux. There are many alternatives on the market.
Outdated or deprecated libraries
These are included here so you know what not to use for your projects.
cImage A simple image processing library for Python. Intended for use in introductory computer science courses. Uses PIL.
PIL Python image library. Hasn’t been updated since 2009, now outdated and insecure. Largely been replaced by Pillow, a friendly fork of PIL.
SimpleCV An open source Python library based on OpenCV designed for rapid development of cmputer vision applications, with accompanying book from Reilly Media. There’s no evidence of continued development, and not compatible with recent versions of Python 3.
File Formats#
For this case study we will be developing techniques to analyze images stored as computer files. Later we may consider applying these techniques to a live video stream, but for now we’ll use stored images.
Things to know about image files.
Raster versus vector images. Image sensors produce raster images.
Image files can get large, and are often stored in some sort of compressed form.
No compression – not commonly encountered since lossless compression is so straightfforward.
Lossless compression – techniques to encode the data using fewer characters. The most common method of storing raw image data.
Lossy compression – willing to sacrifice some image detail for significantly reduced storage requirements. This technology enables jpeg, mpeg, and video streaming protcols.
The suffix of an image file usually (i.e., no guarantee) indicates the image file format.
.svg, .dxf, .eps, .pdf – Vector “document” files which can contain multiple elements in addition to the vector graphics. SVG (scalable vector graphics) is supported by most browsers
.png – The most frequently used losslessly compressed raster image file format widely supported by web browsers. Unlike .jpg, .png allows a transparency channel. It has largely replaces GIF (Graphics Interchange Format) which is now used primarily for simple animations.
.tiff/.tif – Tagged Image File Format was introduced to support cross-platform photo editing. Note that .tif files may encode lossy image formats.
.dng, .raw, and other raw formats – Formats that encode the data directly sampled from the image sensor. Useful for post-processing images, and re-processing images.
.jpeg/.jpg – perhaps the most common standard for image files. This is a lossy format, and can be very lossy in extreme cases.
General recommendations for scientific use.
Use lossless image formats when possible. Store the original as a master file. Use a file naming convention to track derivatives of the original image.
Use .png for line art, graphics, especially for web use.
Use high quality .jpg for photographs and realistic images. Be careful with “generational loss”. Always use sRGB color space which is, by far, the most common color space used for .jpg files.
When possible lossless .tif, .dng, and raw formats to capture and archive original image data.
Image Metadata#
Image files also carry meta-data providing additional information about the image. There several different standards for meta data, the most common being exif data embedded in the image file, or a companion .xmp file hold data in the Extensible Image File Format Metadata Platform format. exiftool is a command line tool included with many operating systems.
Description of Exif file format
import glob
from PIL import Image
from PIL.ExifTags import TAGS
# print list of all files in a directory
path = "./image_data/Jiang Photos/"
files = glob.glob(path + '*')
for file in files:
print(file)
./image_data/Jiang Photos/meso Si Camera Ceta 13000 x 20220311 1544.tif
./image_data/Jiang Photos/meso Si Camera Ceta 21500 x 20220311 1539.tif
./image_data/Jiang Photos/Gold STEM HAADF 59000 x 20220318 1542.tif
./image_data/Jiang Photos/Picture3.tif
./image_data/Jiang Photos/Picture2.tif
# get all files in directory
for file in glob.glob(path + '*'):
# display thumbnail
im_thumb = Image.open(file)
im_thumb.thumbnail((100, 100))
print(f"\n{file}")
display(im_thumb)
im = Image.open(file)
exifdata = im.getexif()
for tag in exifdata.keys():
print(TAGS.get(tag), end=": ")
print(exifdata[tag])
./image_data/Jiang Photos/meso Si Camera Ceta 13000 x 20220311 1544.tif
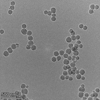
ImageWidth: 1024
ImageLength: 1024
BitsPerSample: (8, 8, 8, 8)
Compression: 1
PhotometricInterpretation: 2
ResolutionUnit: 2
StripOffsets: 8
Orientation: 1
ExtraSamples: 2
SamplesPerPixel: 4
StripByteCounts: 4194304
XResolution: 96.0
YResolution: 96.0
PlanarConfiguration: 1
./image_data/Jiang Photos/meso Si Camera Ceta 21500 x 20220311 1539.tif
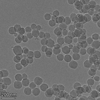
ImageWidth: 1024
ImageLength: 1024
BitsPerSample: (8, 8, 8, 8)
Compression: 1
PhotometricInterpretation: 2
ResolutionUnit: 2
StripOffsets: 8
Orientation: 1
ExtraSamples: 2
SamplesPerPixel: 4
StripByteCounts: 4194304
XResolution: 96.0
YResolution: 96.0
PlanarConfiguration: 1
./image_data/Jiang Photos/Gold STEM HAADF 59000 x 20220318 1542.tif
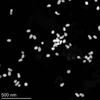
ImageWidth: 2048
ImageLength: 2048
BitsPerSample: (8, 8, 8, 8)
Compression: 1
PhotometricInterpretation: 2
ResolutionUnit: 2
StripOffsets: 8
Orientation: 1
ExtraSamples: 2
SamplesPerPixel: 4
StripByteCounts: 16777216
XResolution: 96.0
YResolution: 96.0
PlanarConfiguration: 1
./image_data/Jiang Photos/Picture3.tif
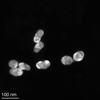
ImageWidth: 613
ImageLength: 613
BitsPerSample: (8, 8, 8, 8)
Compression: 5
PhotometricInterpretation: 2
ResolutionUnit: 2
StripOffsets: (8, 2236, 4452, 6643, 8857, 11100, 13314, 15541, 17764, 19963, 22151, 24364, 26569, 28765, 30986, 33220, 35410, 37602, 39808, 41978, 44146, 46358, 48561, 50768, 52995, 55242, 57444, 59642, 61898, 64093, 66303, 68519, 70703, 72873, 75061, 77234, 79439, 81777, 84235, 86722, 89255, 91781, 94404, 97024, 99583, 102167, 104745, 107338, 109928, 112580, 115197, 117831, 120450, 123003, 125580, 128179, 130796, 133391, 136010, 138567, 141090, 143580, 146079, 148686, 151416, 154143, 156844, 159589, 162382, 165268, 168250, 171242, 174189, 177195, 180312, 183601, 186800, 190157, 193679, 197148, 200512, 203840, 207123, 210363, 213537, 216577, 219496, 222272, 224966, 227690, 230390, 233087, 235784, 238365, 240702, 242922, 245124, 247252, 249448, 251625, 253786, 255934, 258124, 260282, 262432, 264601, 266747, 268872, 271015, 273163, 275353, 277504, 279652, 281811, 283972, 286598, 289451, 292241, 295037, 297147, 299200, 301165, 303264)
ExtraSamples: 2
SamplesPerPixel: 4
RowsPerStrip: 5
StripByteCounts: (2228, 2216, 2191, 2214, 2243, 2214, 2227, 2223, 2199, 2188, 2213, 2205, 2196, 2221, 2234, 2190, 2192, 2206, 2170, 2168, 2212, 2203, 2207, 2227, 2247, 2202, 2198, 2256, 2195, 2210, 2216, 2184, 2170, 2188, 2173, 2205, 2338, 2458, 2487, 2533, 2526, 2623, 2620, 2559, 2584, 2578, 2593, 2590, 2652, 2617, 2634, 2619, 2553, 2577, 2599, 2617, 2595, 2619, 2557, 2523, 2490, 2499, 2607, 2730, 2727, 2701, 2745, 2793, 2886, 2982, 2992, 2947, 3006, 3117, 3289, 3199, 3357, 3522, 3469, 3364, 3328, 3283, 3240, 3174, 3040, 2919, 2776, 2694, 2724, 2700, 2697, 2697, 2581, 2337, 2220, 2202, 2128, 2196, 2177, 2161, 2148, 2190, 2158, 2150, 2169, 2146, 2125, 2143, 2148, 2190, 2151, 2148, 2159, 2161, 2626, 2853, 2790, 2796, 2110, 2053, 1965, 2099, 1385)
XResolution: 150.0
YResolution: 150.0
PlanarConfiguration: 1
Predictor: 2
NewSubfileType: 0
./image_data/Jiang Photos/Picture2.tif
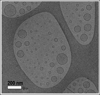
ImageWidth: 685
ImageLength: 651
BitsPerSample: (8, 8, 8, 8)
Compression: 5
PhotometricInterpretation: 2
ResolutionUnit: 2
StripOffsets: (8, 244, 879, 4845, 8872, 12859, 16904, 20928, 24951, 28978, 32980, 36970, 41002, 45056, 49088, 53130, 57196, 61262, 65295, 69340, 73390, 77404, 81421, 85453, 89480, 93477, 97507, 101548, 105587, 109607, 113606, 117626, 121668, 125674, 129694, 133667, 137693, 141669, 145671, 149679, 153676, 157658, 161678, 165669, 169680, 173694, 177703, 181732, 185740, 189740, 193751, 197798, 201842, 205883, 209936, 213972, 217975, 221996, 226031, 230022, 234054, 238105, 242149, 246154, 250165, 254180, 258192, 262231, 266285, 270353, 274340, 278373, 282372, 286393, 290425, 294449, 298539, 302574, 306592, 310609, 314630, 318611, 322653, 326620, 330608, 334617, 338622, 342624, 346602, 350616, 354666, 358663, 362659, 366683, 370701, 374709, 378705, 382722, 386709, 390714, 394693, 398683, 402676, 406712, 410736, 414742, 418738, 422743, 426770, 430788, 434821, 438838, 442849, 446857, 450829, 454811, 458819, 462879, 466867, 470882, 474876, 478870, 482876, 486891, 490879, 494837, 498861, 502881, 506866, 510862, 514832, 518801, 522816, 526833, 530875, 534889, 538913, 542915, 546897, 550942, 555008, 559226, 563412, 567660, 571872, 576022, 580126, 584140, 587776, 591397, 595043, 598632, 602260, 606062, 610094, 614096, 618123, 622152, 626163, 630141, 634131, 635381, 635621)
ExtraSamples: 2
SamplesPerPixel: 4
RowsPerStrip: 4
StripByteCounts: (236, 635, 3966, 4027, 3987, 4045, 4024, 4023, 4027, 4002, 3990, 4032, 4054, 4032, 4042, 4066, 4066, 4033, 4045, 4050, 4014, 4017, 4032, 4027, 3997, 4030, 4041, 4039, 4020, 3999, 4020, 4042, 4006, 4020, 3973, 4026, 3976, 4002, 4008, 3997, 3982, 4020, 3991, 4011, 4014, 4009, 4029, 4008, 4000, 4011, 4047, 4044, 4041, 4053, 4036, 4003, 4021, 4035, 3991, 4032, 4051, 4044, 4005, 4011, 4015, 4012, 4039, 4054, 4068, 3987, 4033, 3999, 4021, 4032, 4024, 4090, 4035, 4018, 4017, 4021, 3981, 4042, 3967, 3988, 4009, 4005, 4002, 3978, 4014, 4050, 3997, 3996, 4024, 4018, 4008, 3996, 4017, 3987, 4005, 3979, 3990, 3993, 4036, 4024, 4006, 3996, 4005, 4027, 4018, 4033, 4017, 4011, 4008, 3972, 3982, 4008, 4060, 3988, 4015, 3994, 3994, 4006, 4015, 3988, 3958, 4024, 4020, 3985, 3996, 3970, 3969, 4015, 4017, 4042, 4014, 4024, 4002, 3982, 4045, 4066, 4218, 4186, 4248, 4212, 4150, 4104, 4014, 3636, 3621, 3646, 3589, 3628, 3802, 4032, 4002, 4027, 4029, 4011, 3978, 3990, 1250, 240, 194)
XResolution: 150.0
YResolution: 150.0
PlanarConfiguration: 1
Predictor: 2
NewSubfileType: 0
More Examples of Image Metadata#
im = Image.open("image_data/25-miniM.tif")
exifdata = im.getexif()
for tag in exifdata.keys():
print(TAGS.get(tag), end=": ")
print(exifdata[tag])
ImageWidth: 2560
ImageLength: 2048
BitsPerSample: (8, 8, 8)
Compression: 7
PhotometricInterpretation: 2
ResolutionUnit: 2
StripOffsets: (8, 6667, 13846, 21234, 28748, 36663, 44541, 52257, 59920, 68103, 76470, 84801, 92967, 101065, 109291, 117325, 125566, 134051, 142370, 150906, 159120, 167870, 176525, 184896, 193550, 202599, 212175, 221421, 230888, 240234, 249583, 259208, 268703, 278555, 287858, 297116, 306591, 315868, 324932, 333906, 343389, 353574, 363655, 373182, 382338, 391623, 401414, 411103, 420572, 430054, 439685, 449544, 459430, 469392, 479530, 489117, 499277, 509085, 518800, 529070, 538960, 548798, 558998, 568930, 578965, 589126, 599742, 609597, 619633, 629830, 639515, 649251, 659597, 670179, 680309, 690235, 700715, 711300, 721743, 732228, 742695, 753030, 763196, 773749, 784125, 794607, 805237, 815773, 825899, 836277, 846689, 857294, 868346, 879050, 889660, 899919, 910285, 920860, 931897, 942666, 953252, 963478, 973771, 984458, 995721, 1006437, 1016633, 1027051, 1037482, 1048445, 1059569, 1071010, 1082087, 1092997, 1103840, 1114316, 1124415, 1135219, 1146815, 1157687, 1168222, 1178620, 1188775, 1199535, 1211301, 1222922, 1233899, 1244777, 1255511, 1265916, 1277261, 1288636, 1299877, 1310418, 1320906, 1331169, 1342329, 1353676, 1364849, 1375510, 1385825, 1395986, 1406570, 1417437, 1429291, 1440536, 1450494, 1461036, 1471250, 1481621, 1492792, 1504148, 1514875, 1525178, 1535520, 1545933, 1556765, 1568268, 1579027, 1589235, 1599418, 1609475, 1620241, 1631447, 1641986, 1652345, 1662203, 1672131, 1682461, 1693317, 1703962, 1714585, 1724696, 1734535, 1744893, 1755437, 1766417, 1776993, 1787250, 1797313, 1807142, 1817607, 1827969, 1838234, 1848133, 1857742, 1867580, 1877615, 1888275, 1898686, 1908491, 1918552, 1928352, 1937853, 1948071, 1958372, 1968341, 1978085, 1987887, 1997251, 2007066, 2017031, 2026518, 2035897, 2045357, 2054511, 2064623, 2074879, 2084801, 2094310, 2103625, 2113117, 2122695, 2132596, 2142348, 2152178, 2161696, 2171005, 2180531, 2190177, 2200015, 2209322, 2218759, 2227730, 2237213, 2246795, 2255984, 2265281, 2274505, 2283670, 2292756, 2301922, 2311191, 2320216, 2329073, 2337990, 2347398, 2356706, 2365873, 2374919, 2384239, 2393509, 2402604, 2411839, 2421517, 2431270, 2441758, 2451511, 2460555, 2468861, 2476702, 2484576, 2492080, 2499378, 2506042, 2513116)
Orientation: 1
JPEGTables: b'\xff\xd8\xff\xdb\x00C\x00\x03\x02\x02\x03\x02\x02\x03\x03\x03\x03\x04\x03\x03\x04\x05\x08\x05\x05\x04\x04\x05\n\x07\x07\x06\x08\x0c\n\x0c\x0c\x0b\n\x0b\x0b\r\x0e\x12\x10\r\x0e\x11\x0e\x0b\x0b\x10\x16\x10\x11\x13\x14\x15\x15\x15\x0c\x0f\x17\x18\x16\x14\x18\x12\x14\x15\x14\xff\xc4\x00\x1f\x00\x00\x01\x05\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x01\x02\x03\x04\x05\x06\x07\x08\t\n\x0b\xff\xc4\x00\xb5\x10\x00\x02\x01\x03\x03\x02\x04\x03\x05\x05\x04\x04\x00\x00\x01}\x01\x02\x03\x00\x04\x11\x05\x12!1A\x06\x13Qa\x07"q\x142\x81\x91\xa1\x08#B\xb1\xc1\x15R\xd1\xf0$3br\x82\t\n\x16\x17\x18\x19\x1a%&\'()*456789:CDEFGHIJSTUVWXYZcdefghijstuvwxyz\x83\x84\x85\x86\x87\x88\x89\x8a\x92\x93\x94\x95\x96\x97\x98\x99\x9a\xa2\xa3\xa4\xa5\xa6\xa7\xa8\xa9\xaa\xb2\xb3\xb4\xb5\xb6\xb7\xb8\xb9\xba\xc2\xc3\xc4\xc5\xc6\xc7\xc8\xc9\xca\xd2\xd3\xd4\xd5\xd6\xd7\xd8\xd9\xda\xe1\xe2\xe3\xe4\xe5\xe6\xe7\xe8\xe9\xea\xf1\xf2\xf3\xf4\xf5\xf6\xf7\xf8\xf9\xfa\xff\xd9'
SamplesPerPixel: 3
RowsPerStrip: 8
StripByteCounts: (6659, 7179, 7388, 7514, 7915, 7878, 7716, 7663, 8183, 8367, 8331, 8166, 8098, 8226, 8034, 8241, 8485, 8319, 8536, 8214, 8750, 8655, 8371, 8654, 9049, 9576, 9246, 9467, 9346, 9349, 9625, 9495, 9852, 9303, 9258, 9475, 9277, 9064, 8974, 9483, 10185, 10081, 9527, 9156, 9285, 9791, 9689, 9469, 9482, 9631, 9859, 9886, 9962, 10138, 9587, 10160, 9808, 9715, 10270, 9890, 9838, 10200, 9932, 10035, 10161, 10616, 9855, 10036, 10197, 9685, 9736, 10346, 10582, 10130, 9926, 10480, 10585, 10443, 10485, 10467, 10335, 10166, 10553, 10376, 10482, 10630, 10536, 10126, 10378, 10412, 10605, 11052, 10704, 10610, 10259, 10366, 10575, 11037, 10769, 10586, 10226, 10293, 10687, 11263, 10716, 10196, 10418, 10431, 10963, 11124, 11441, 11077, 10910, 10843, 10476, 10099, 10804, 11596, 10872, 10535, 10398, 10155, 10760, 11766, 11621, 10977, 10878, 10734, 10405, 11345, 11375, 11241, 10541, 10488, 10263, 11160, 11347, 11173, 10661, 10315, 10161, 10584, 10867, 11854, 11245, 9958, 10542, 10214, 10371, 11171, 11356, 10727, 10303, 10342, 10413, 10832, 11503, 10759, 10208, 10183, 10057, 10766, 11206, 10539, 10359, 9858, 9928, 10330, 10856, 10645, 10623, 10111, 9839, 10358, 10544, 10980, 10576, 10257, 10063, 9829, 10465, 10362, 10265, 9899, 9609, 9838, 10035, 10660, 10411, 9805, 10061, 9800, 9501, 10218, 10301, 9969, 9744, 9802, 9364, 9815, 9965, 9487, 9379, 9460, 9154, 10112, 10256, 9922, 9509, 9315, 9492, 9578, 9901, 9752, 9830, 9518, 9309, 9526, 9646, 9838, 9307, 9437, 8971, 9483, 9582, 9189, 9297, 9224, 9165, 9086, 9166, 9269, 9025, 8857, 8917, 9408, 9308, 9167, 9046, 9320, 9270, 9095, 9235, 9678, 9753, 10488, 9753, 9044, 8306, 7841, 7874, 7504, 7298, 6664, 7074, 6721)
XResolution: 300.0
YResolution: 300.0
PlanarConfiguration: 1
NewSubfileType: 0
!/usr/local/bin/exiftool image_data/25-miniM.tif
ExifTool Version Number : 11.52
File Name : 25-miniM.tif
Directory : image_data
File Size : 2.4 MB
File Modification Date/Time : 2021:11:23 13:25:31-05:00
File Access Date/Time : 2022:11:16 17:54:05-05:00
File Inode Change Date/Time : 2022:11:16 17:54:03-05:00
File Permissions : rw-r--r--
File Type : TIFF
File Type Extension : tif
MIME Type : image/tiff
Exif Byte Order : Little-endian (Intel, II)
Subfile Type : Full-resolution Image
Image Width : 2560
Image Height : 2048
Bits Per Sample : 8 8 8
Compression : JPEG
Photometric Interpretation : RGB
Strip Offsets : (Binary data 1924 bytes, use -b option to extract)
Orientation : Horizontal (normal)
Samples Per Pixel : 3
Rows Per Strip : 8
Strip Byte Counts : (Binary data 1405 bytes, use -b option to extract)
X Resolution : 300
Y Resolution : 300
Planar Configuration : Chunky
Resolution Unit : inches
JPEG Tables : (Binary data 289 bytes, use -b option to extract)
Image Size : 2560x2048
Megapixels : 5.2
What’s not present in this example? (Hint: What colors are being displayed?)
Here’s the exif data for a photograph prepared through a typical photographer’s workflow: RAW –> Noise Reduction –> Adobe Lightroom –> .jpg for export. Carefully look for the color space data.
im = Image.open("image_data/eagles-photo-low-quality.jpg")
exifdata = im.getexif()
for tag in exifdata.keys():
print(TAGS.get(tag), end=": ")
print(exifdata[tag])
print()
!/usr/local/bin/exiftool image_data/eagles-photo-low-quality.jpg
GPSInfo: 926
ResolutionUnit: 2
ExifOffset: 246
Make: OLYMPUS CORPORATION
Model: E-M1X
Software: Adobe Lightroom 5.0 (Macintosh)
DateTime: 2021:11:29 16:25:58
Copyright: © Jeffrey Kantor
XResolution: 240.0
YResolution: 240.0
ExifTool Version Number : 11.52
File Name : eagles-photo-low-quality.jpg
Directory : image_data
File Size : 248 kB
File Modification Date/Time : 2021:11:29 16:25:58-05:00
File Access Date/Time : 2022:11:16 17:54:14-05:00
File Inode Change Date/Time : 2022:11:16 17:54:03-05:00
File Permissions : rw-r--r--
File Type : JPEG
File Type Extension : jpg
MIME Type : image/jpeg
Exif Byte Order : Little-endian (Intel, II)
Make : OLYMPUS CORPORATION
Camera Model Name : E-M1X
X Resolution : 240
Y Resolution : 240
Resolution Unit : inches
Software : Adobe Lightroom 5.0 (Macintosh)
Modify Date : 2021:11:29 16:25:58
Copyright : © Jeffrey Kantor
Exposure Time : 1/400
F Number : 8.0
Exposure Program : Aperture-priority AE
ISO : 500
Sensitivity Type : Standard Output Sensitivity
Exif Version : 0231
Date/Time Original : 2021:07:15 07:54:21
Create Date : 2021:07:15 07:54:21
Offset Time : -05:00
Shutter Speed Value : 1/400
Aperture Value : 8.0
Exposure Compensation : -0.3
Max Aperture Value : 8.0
Subject Distance : 35.4 m
Metering Mode : Multi-segment
Light Source : Unknown
Flash : Off, Did not fire
Focal Length : 600.0 mm
Color Space : sRGB
Focal Plane X Resolution : 3010.362305
Focal Plane Y Resolution : 3010.362305
Focal Plane Resolution Unit : cm
File Source : Digital Camera
CFA Pattern : [Red,Green][Green,Blue]
Custom Rendered : Normal
Exposure Mode : Manual
White Balance : Auto
Digital Zoom Ratio : 1
Focal Length In 35mm Format : 1202 mm
Scene Capture Type : Standard
Gain Control : High gain up
Contrast : Normal
Saturation : Normal
Sharpness : Normal
Serial Number : BJ4A06646
Lens Info : 600mm f/8
Lens Model : M.300mm F4.0 + MC-20
Lens Serial Number : ACA204117
GPS Version ID : 2.2.0.0
GPS Img Direction : 134.5
Compression : JPEG (old-style)
Thumbnail Offset : 1070
Thumbnail Length : 19508
Displayed Units X : inches
Displayed Units Y : inches
Current IPTC Digest : 786d1b99d5d40bcdba895d751f9372b7
Coded Character Set : UTF8
Application Record Version : 4
Time Created : 07:54:21
Digital Creation Date : 2021:07:15
Digital Creation Time : 07:54:21
Copyright Notice : © Jeffrey Kantor
Photoshop Thumbnail : (Binary data 19508 bytes, use -b option to extract)
IPTC Digest : 786d1b99d5d40bcdba895d751f9372b7
Profile CMM Type : Linotronic
Profile Version : 2.1.0
Profile Class : Display Device Profile
Color Space Data : RGB
Profile Connection Space : XYZ
Profile Date Time : 1998:02:09 06:49:00
Profile File Signature : acsp
Primary Platform : Microsoft Corporation
CMM Flags : Not Embedded, Independent
Device Manufacturer : Hewlett-Packard
Device Model : sRGB
Device Attributes : Reflective, Glossy, Positive, Color
Rendering Intent : Perceptual
Connection Space Illuminant : 0.9642 1 0.82491
Profile Creator : Hewlett-Packard
Profile ID : 0
Profile Copyright : Copyright (c) 1998 Hewlett-Packard Company
Profile Description : sRGB IEC61966-2.1
Media White Point : 0.95045 1 1.08905
Media Black Point : 0 0 0
Red Matrix Column : 0.43607 0.22249 0.01392
Green Matrix Column : 0.38515 0.71687 0.09708
Blue Matrix Column : 0.14307 0.06061 0.7141
Device Mfg Desc : IEC http://www.iec.ch
Device Model Desc : IEC 61966-2.1 Default RGB colour space - sRGB
Viewing Cond Desc : Reference Viewing Condition in IEC61966-2.1
Viewing Cond Illuminant : 19.6445 20.3718 16.8089
Viewing Cond Surround : 3.92889 4.07439 3.36179
Viewing Cond Illuminant Type : D50
Luminance : 76.03647 80 87.12462
Measurement Observer : CIE 1931
Measurement Backing : 0 0 0
Measurement Geometry : Unknown
Measurement Flare : 0.999%
Measurement Illuminant : D65
Technology : Cathode Ray Tube Display
Red Tone Reproduction Curve : (Binary data 2060 bytes, use -b option to extract)
Green Tone Reproduction Curve : (Binary data 2060 bytes, use -b option to extract)
Blue Tone Reproduction Curve : (Binary data 2060 bytes, use -b option to extract)
XMP Toolkit : Adobe XMP Core 7.0-c000 1.000000, 0000/00/00-00:00:00
White Level : 29222
Adobe White Level : 29944
Creator Tool : Adobe Lightroom 5.0 (Macintosh)
Rating : 4
Metadata Date : 2021:11:29 16:25:58-05:00
Lens : M.300mm F4.0 + MC-20
Approximate Focus Distance : 35.4
Flash Compensation : 0
Date Created : 2021:07:15 07:54:21
Document ID : xmp.did:35e88d02-dcad-40ee-954c-d74068c1e965
Original Document ID : DD64D26CAFF63683666FACABB6CE7C1D
Instance ID : xmp.iid:35e88d02-dcad-40ee-954c-d74068c1e965
Format : image/jpeg
Raw File Name :
Version : 14.0
Process Version : 11.0
Color Temperature : 4926
Tint : +15
Exposure 2012 : +0.03
Contrast 2012 : +33
Highlights 2012 : -66
Shadows 2012 : +5
Whites 2012 : +2
Blacks 2012 : -21
Texture : +40
Clarity 2012 : +13
Dehaze : +8
Vibrance : +20
Parametric Shadows : 0
Parametric Darks : 0
Parametric Lights : 0
Parametric Highlights : 0
Parametric Shadow Split : 25
Parametric Midtone Split : 50
Parametric Highlight Split : 75
Sharpen Radius : +1.0
Sharpen Detail : 25
Sharpen Edge Masking : 0
Luminance Smoothing : 0
Color Noise Reduction : 0
Hue Adjustment Red : 0
Hue Adjustment Orange : 0
Hue Adjustment Yellow : 0
Hue Adjustment Green : 0
Hue Adjustment Aqua : 0
Hue Adjustment Blue : 0
Hue Adjustment Purple : 0
Hue Adjustment Magenta : 0
Saturation Adjustment Red : 0
Saturation Adjustment Orange : 0
Saturation Adjustment Yellow : 0
Saturation Adjustment Green : 0
Saturation Adjustment Aqua : 0
Saturation Adjustment Blue : 0
Saturation Adjustment Purple : 0
Saturation Adjustment Magenta : 0
Luminance Adjustment Red : 0
Luminance Adjustment Orange : 0
Luminance Adjustment Yellow : 0
Luminance Adjustment Green : 0
Luminance Adjustment Aqua : 0
Luminance Adjustment Blue : 0
Luminance Adjustment Purple : 0
Luminance Adjustment Magenta : 0
Split Toning Shadow Hue : 0
Split Toning Shadow Saturation : 0
Split Toning Highlight Hue : 0
Split Toning Highlight Saturation: 0
Split Toning Balance : 0
Color Grade Midtone Hue : 0
Color Grade Midtone Sat : 0
Color Grade Shadow Lum : 0
Color Grade Midtone Lum : 0
Color Grade Highlight Lum : 0
Color Grade Blending : 50
Color Grade Global Hue : 0
Color Grade Global Sat : 0
Color Grade Global Lum : 0
Auto Lateral CA : 0
Lens Profile Enable : 0
Lens Manual Distortion Amount : 0
Vignette Amount : 0
Defringe Purple Amount : 0
Defringe Purple Hue Lo : 30
Defringe Purple Hue Hi : 70
Defringe Green Amount : 0
Defringe Green Hue Lo : 40
Defringe Green Hue Hi : 60
Perspective Upright : 0
Perspective Vertical : 0
Perspective Horizontal : 0
Perspective Rotate : 0.0
Perspective Aspect : 0
Perspective Scale : 100
Perspective X : 0.00
Perspective Y : 0.00
Grain Amount : 0
Post Crop Vignette Amount : 0
Shadow Tint : 0
Red Hue : 0
Red Saturation : 0
Green Hue : 0
Green Saturation : 0
Blue Hue : 0
Blue Saturation : 0
Override Look Vignette : False
Tone Curve Name 2012 : Linear
Camera Profile : Adobe Standard
Camera Profile Digest : 17201179F5294A1E72C1552BAE550EEF
Auto Tone Digest : 1715A4BED36C368980DA388E7373CCEE
Auto Tone Digest No Sat : 3E753A3B412B959232482F410AEDEFE8
Has Settings : True
Crop Top : 0.078092
Crop Left : 0.170981
Crop Bottom : 0.956094
Crop Right : 0.957141
Crop Angle : 0.192816
Crop Constrain To Warp : 0
Has Crop : True
Already Applied : True
History Action : edited, derived, saved
History When : 2021:07:15 22:11:47Z, 2021:11:29 16:25:58-05:00
History Parameters : converted from image/dng to image/jpeg, saved to new location
History Instance ID : xmp.iid:35e88d02-dcad-40ee-954c-d74068c1e965
History Software Agent : Adobe Lightroom 5.0 (Macintosh)
History Changed : /
Derived From Document ID : DD64D26CAFF63683666FACABB6CE7C1D
Derived From Original Document ID: DD64D26CAFF63683666FACABB6CE7C1D
Rights : © Jeffrey Kantor
Tone Curve PV2012 : 0, 0, 255, 255
Tone Curve PV2012 Red : 0, 0, 255, 255
Tone Curve PV2012 Green : 0, 0, 255, 255
Tone Curve PV2012 Blue : 0, 0, 255, 255
Look Name : Adobe Portrait
Look Amount : 1
Look Uuid : D6496412E06A83789C499DF9540AA616
Look Supports Amount : false
Look Supports Monochrome : false
Look Supports Output Referred : false
Look Group : Profiles
Look Parameters Version : 14.0
Look Parameters Process Version : 11.0
Look Parameters Convert To Grayscale: False
Look Parameters Camera Profile : Adobe Standard
Look Parameters Look Table : E5A76DBB8B3F132A04C01AF45DC2EF1B
Look Parameters Tone Curve PV2012: 0, 0, 66, 64, 190, 192, 255, 255
DCT Encode Version : 100
APP14 Flags 0 : [14], Encoded with Blend=1 downsampling
APP14 Flags 1 : (none)
Color Transform : YCbCr
Image Width : 1704
Image Height : 2048
Encoding Process : Baseline DCT, Huffman coding
Bits Per Sample : 8
Color Components : 3
Y Cb Cr Sub Sampling : YCbCr4:2:0 (2 2)
Aperture : 8.0
Date/Time Created : 2021:07:15 07:54:21
Digital Creation Date/Time : 2021:07:15 07:54:21
Image Size : 1704x2048
Megapixels : 3.5
Scale Factor To 35 mm Equivalent: 2.0
Shutter Speed : 1/400
Modify Date : 2021:11:29 16:25:58-05:00
Thumbnail Image : (Binary data 19508 bytes, use -b option to extract)
Circle Of Confusion : 0.015 mm
Depth Of Field : 0.82 m (34.99 - 35.82 m)
Field Of View : 1.7 deg
Focal Length : 600.0 mm (35 mm equivalent: 1202.0 mm)
Hyperfocal Distance : 3000.37 m
Light Value : 12.3
Calibrating Monitors#
Accurate color reproduction requires color management from source to display. At each Color management is now a standard part of most operating systems